Overview of Python Imaging Library (PIL) Image Class Wrapper
Recently I have been working in Image Processing using Python. So I am posting my about what I learn during my work. You can also find the complete notebooks at https://github.com/opnchaudhary/python-notebooks . I will be doing the basic operations related to Image manipulation. For your better understanding I have put comments in each lines.
Lets get started:
This is a very simple example which opens the image file, 'image.png' and prints the format, size and mode of the image. The last line displays the image. The following is the sample Image I used in my tests.
Another example to convert image formats:
Cropping image:
Converting modes of image (example is chaing RGB image to Gray Scale)
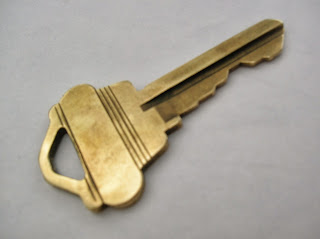
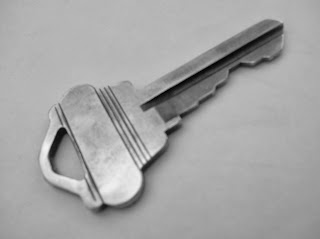
RGB Mode L Mode
Transpose operations
You can also use:
Lets get started:
import Image #import Image library
img=Image.open('image.png') #load an image using the Image library
print img.format,img.size,img.mode #print the format,size and mode
img.show()#display the image
This is a very simple example which opens the image file, 'image.png' and prints the format, size and mode of the image. The last line displays the image. The following is the sample Image I used in my tests.
Another example to convert image formats:
import Image #import Image library
img=Image.open('image.png') #load an image using the Image libary
img.save('newimage.jpg',"JPEG")#save the image in jpeg format
Creating a thumbnail:import Image #import Image library
img=Image.open('image.png') #load an image using the Image libary
img.thumbnail((128, 128))#create thumbnail image of the specified size
img.show()#display the image
Cropping image:
import Image #import Image library
img=Image.open('image.png') #load an image using the Image libary
box = (150, 150, 400, 400) #crop area (left, upper, right, lower)
region = img.crop(box)#crop the image
region.show()#display the cropped region
Converting modes of image (example is chaing RGB image to Gray Scale)
import Image #import Image library
img=Image.open('key.jpg') #load an image using the Image libary
print img.mode
img=img.convert("L") #convert to L mode
print img.mode
img.show()
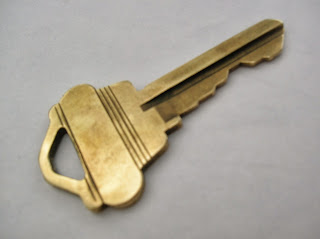
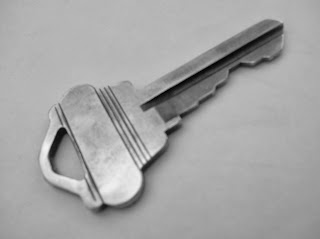
RGB Mode L Mode
Transpose operations
import Image #import Image library
img=Image.open('image.png') #load an image using the Image libary
img=img.rotate(45)#rotate by 45 degree
img.show()#display the image
You can also use:
img=img.transpose(Image.FLIP_LEFT_RIGHT)#flip horizontal
img=img.transpose(Image.FLIP_TOP_BOTTOM)#flip vertical
You may also try various filters over the image:
import Image,ImageFilter #import Image Library
img=Image.open('image.png')
img=img.filter(ImageFilter.EDGE_ENHANCE)#apply EDGE_ENHANCE filter
img.show();
Some other filters you may want to play with are DETAIL,CONTOUR, FIND_EDGES,GaussianBlur,EDGE_ENHANCE
Comments
Post a Comment