Automate file upload in Selenium IDE
Selenium IDE is an integrated development environment for Selenium tests. It records your interactions with websites to help you generate and maintain site automation, tests, and remove the need to manually step through repetitive tasks. Selenium IDE can be installed in Google Chrome from here. For Firefox visit this link.
File upload in Selenium IDE cannot be automated simply by recording from the IDE. Because when we select the file input field, the dialog that opens up is a system window which cannot be controlled by Selenium IDE. Therefore, clicking the input file field to select a file will not work. Also the selected file path is changed to C:\fakepath\filename.extension. So to fix this issue, we need to configure the browser to support file upload and then instead of clicking the input field we set the file using ‘type’ selenium command which is shown below.
First, to support file upload in Selenium IDE, configure your browser to support file upload:
- Visit ‘chrome://extensions/’
- Click ‘Detail’ on Selenium IDE extension.
- Enable ‘Allow access to file URLs’
Let’s take a simple file upload application for our test case which I have copied from tutorialspoint.com. This is a file upload code in PHP.
<?php
if(isset($_FILES['image'])){
$errors= array();
$file_name = $_FILES['image']['name'];
$file_size =$_FILES['image']['size'];
$file_tmp =$_FILES['image']['tmp_name'];
$file_type=$_FILES['image']['type'];
$file_ext=strtolower(end(explode('.',$_FILES['image']['name'])));
$extensions= array("jpeg","jpg","png");
if(in_array($file_ext,$extensions)=== false){
$errors[]="extension not allowed, please choose a JPEG or PNG file.";
}
if($file_size > 2097152){
$errors[]='File size must be excately 2 MB';
}
if(empty($errors)==true){
move_uploaded_file($file_tmp,"images/".$file_name);
echo "Success";
}else{
print_r($errors);
}
}
?>
<html>
<body>
<form action="" method="POST" enctype="multipart/form-data">
<input type="file" name="image" />
<input type="submit"/>
</form>
</body>
</html>
Now let’s create a selenium test as follows.
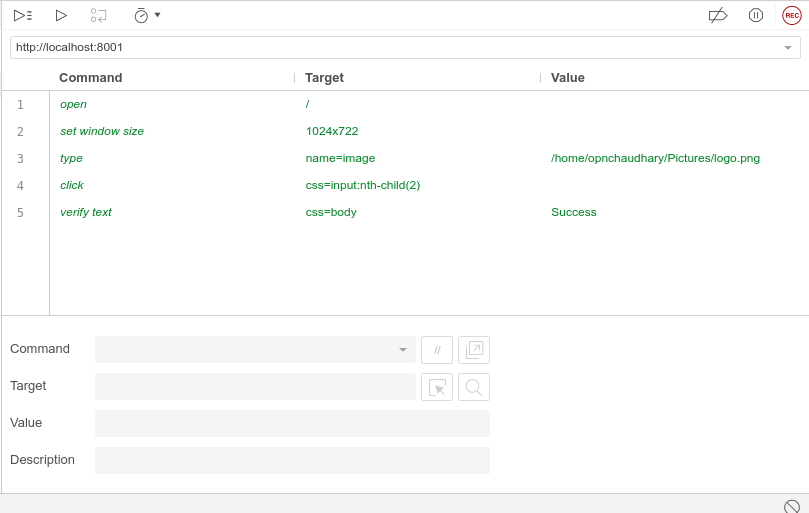
The content inside the selenium test script is as follows.
{
“id”: “6bd52498–446e-4649-bec4–7229881e059b”,
“version”: “2.0”,
“name”: “Test”,
“url”: “http://localhost:8001",
“tests”: [{
“id”: “052db9ee-6734–461f-9131–1aff48478570”,
“name”: “File upload”,
“commands”: [{
“id”: “24ae2a66–8783–447c-9491–6dd79418b713”,
“comment”: “”,
“command”: “open”,
“target”: “/”,
“targets”: [],
“value”: “”
}, {
“id”: “bd444616-a164–433f-a11e-c45815030bc5”,
“comment”: “”,
“command”: “setWindowSize”,
“target”: “1024x722”,
“targets”: [],
“value”: “”
}, {
“id”: “a9440ecf-938d-49a2-bf8c-917ecef2916d”,
“comment”: “”,
“command”: “click”,
“target”: “name=image”,
“targets”: [
[“name=image”, “name”],
[“css=input:nth-child(1)”, “css:finder”],
[“xpath=//input[@name=’image’]”, “xpath:attributes”],
[“xpath=//input”, “xpath:position”]
],
“value”: “”
}, {
“id”: “aadea3b4-be18–43d3–97dd-efb3dea53470”,
“comment”: “”,
“command”: “sendKeys”,
“target”: “name=image”,
“targets”: [],
“value”: “/home/opnchaudhary/Pictures/logo.png”
}, {
“id”: “ac202119–398e-427a-a862-a7b799e69817”,
“comment”: “”,
“command”: “click”,
“target”: “css=input:nth-child(2)”,
“targets”: [
[“css=input:nth-child(2)”, “css:finder”],
[“xpath=//input[@type=’submit’]”, “xpath:attributes”],
[“xpath=//input[2]”, “xpath:position”]
],
“value”: “”
}, {
“id”: “8e0d5e88-f329–4365–8547–402b16f1986f”,
“comment”: “”,
“command”: “verifyText”,
“target”: “css=body”,
“targets”: [
[“css=body”, “css:finder”],
[“xpath=//body”, “xpath:position”]
],
“value”: “Success”
}]
}],
“suites”: [{
“id”: “c05f72c7–4f6c-4c2f-b150–88c3a001454b”,
“name”: “Default Suite”,
“persistSession”: false,
“parallel”: false,
“timeout”: 300,
“tests”: [“052db9ee-6734–461f-9131–1aff48478570”]
}],
“urls”: [“http://localhost:8001/”],
“plugins”: []
}
“id”: “6bd52498–446e-4649-bec4–7229881e059b”,
“version”: “2.0”,
“name”: “Test”,
“url”: “http://localhost:8001",
“tests”: [{
“id”: “052db9ee-6734–461f-9131–1aff48478570”,
“name”: “File upload”,
“commands”: [{
“id”: “24ae2a66–8783–447c-9491–6dd79418b713”,
“comment”: “”,
“command”: “open”,
“target”: “/”,
“targets”: [],
“value”: “”
}, {
“id”: “bd444616-a164–433f-a11e-c45815030bc5”,
“comment”: “”,
“command”: “setWindowSize”,
“target”: “1024x722”,
“targets”: [],
“value”: “”
}, {
“id”: “a9440ecf-938d-49a2-bf8c-917ecef2916d”,
“comment”: “”,
“command”: “click”,
“target”: “name=image”,
“targets”: [
[“name=image”, “name”],
[“css=input:nth-child(1)”, “css:finder”],
[“xpath=//input[@name=’image’]”, “xpath:attributes”],
[“xpath=//input”, “xpath:position”]
],
“value”: “”
}, {
“id”: “aadea3b4-be18–43d3–97dd-efb3dea53470”,
“comment”: “”,
“command”: “sendKeys”,
“target”: “name=image”,
“targets”: [],
“value”: “/home/opnchaudhary/Pictures/logo.png”
}, {
“id”: “ac202119–398e-427a-a862-a7b799e69817”,
“comment”: “”,
“command”: “click”,
“target”: “css=input:nth-child(2)”,
“targets”: [
[“css=input:nth-child(2)”, “css:finder”],
[“xpath=//input[@type=’submit’]”, “xpath:attributes”],
[“xpath=//input[2]”, “xpath:position”]
],
“value”: “”
}, {
“id”: “8e0d5e88-f329–4365–8547–402b16f1986f”,
“comment”: “”,
“command”: “verifyText”,
“target”: “css=body”,
“targets”: [
[“css=body”, “css:finder”],
[“xpath=//body”, “xpath:position”]
],
“value”: “Success”
}]
}],
“suites”: [{
“id”: “c05f72c7–4f6c-4c2f-b150–88c3a001454b”,
“name”: “Default Suite”,
“persistSession”: false,
“parallel”: false,
“timeout”: 300,
“tests”: [“052db9ee-6734–461f-9131–1aff48478570”]
}],
“urls”: [“http://localhost:8001/”],
“plugins”: []
}
Currently file upload works only in Google Chrome.
Comments
Post a Comment